You can have pointers to structured data types, just like you can point to any other type, for example
struct customer * cust_pointer ;
This is a pointer to a customer record. You would set it to point somewhere in the usual way :
cust_pointer = &OnlyOne ;
If you think about it, we must be able to use pointers to structures because we can have arrays of structures and we know that an array is simply a lump of memory of the appropriate size, with a pointer to the bottom of it.
C keeps track of pointer types for us. This is particularly important when we start modifying the value of a pointer, for example :
cust_pointer++ ;
This means increase the value of cust_pointer by one, i.e. make cust_pointer point to the next customer record in memory. C knows how many memory locations that a customer record takes, so it moves down memory that amount.
When we have used a pointer before we have used a * to de-reference it. De-reference means get me the thing that this pointer points at so that :
*cust_pointer
- means the structure pointed at by cust_pointer. When you want to get hold of one of the members of a structure which you are pointing at you have to tell C to de-reference the pointer to the structure and then return the required member. You do this by replacing the . between a structured variable and a member name with a -> between the pointer and the member name. The -> is made up of the character minus (-) directly followed by the character greater than (>)
cust_pointer->balance = 0 ;
- would set the balance of the customer pointed at by cust_pointer to 0.
You can use pointers to structures to create linked lists. In these each item in the list holds a pointer to the next. This technique is sometimes used in preference to creating an array of structures, since with a linked list you only need to use as much storage as is required, rather than grabbing the maximum amount of space as with an array. On the right you can see how this would work. I have created a link list of customer items. The structure definition holds a pointer to customers as the last field in the structure.
Note how the last item in the list has its pointer set to NULL, so that my program can tell when it has reached the end of the list. I would have to have a pointer to the start of the list as well.
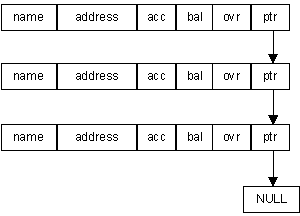
struct customer { char name [NAME_LENGTH] ; char address [ADDR_LENGTH] ; int account ; int balance ; int overdraft ; struct customer * ptr ; } ;